Vue Js Divide Two Numbers:In this tutorial, we will demonstrate how to create a basic Vue.js application to divide two numbers. We’ll provide you with an example code, step-by-step explanations, and the corresponding output
To begin, let’s take a look at the HTML and Vue.js code that creates our “Divide Two Numbers” application:
In this code, we define a Vue.js app with three data properties: num1
, num2
, and result
. The divideNumbers
method calculates the division result and handles division by zero, displaying an error message when necessary.
Vue Js Divide Two Numbers Example
<div id="app">
<h3>Vue Js Divide Two Numbers</h3>
<div>
Enter the first number: <input v-model="num1" type="number" />
</div>
<div>
Enter the second number: <input v-model="num2" type="number" />
</div>
<div>
<button @click="divideNumbers">Divide</button>
</div>
<p>
Result of division: {{ result }}
</p>
</div>
In this JavaScript code, we define a Vue.js app with data properties num1
, num2
, and result
. The divideNumbers
method calculates the division result and handles division by zero, displaying an error message when necessary.
Javascript Code
<script type="module">
const app = Vue.createApp({
data() {
return {
num1: 12,
num2: 7,
result: null,
};
},
methods: {
divideNumbers() {
if (this.num2 === 0) {
this.result = "Cannot divide by zero";
} else {
this.result = this.num1 / this.num2;
}
},
},
});
app.mount("#app");
</script>
Output of Vue.js Divide Two Numbers
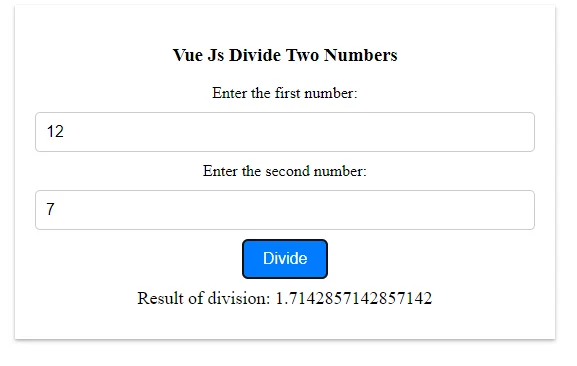
When you open this Vue.js application in your browser, you will see the user interface with input fields for two numbers and a “Divide” button. If you enter valid numbers and click the button, the result of the division will be displayed below.
If you attempt to divide by zero, you will receive a “Cannot divide by zero” error message.
This simple Vue.js application demonstrates the basic principles of data binding and event handling, making it a great starting point for more complex Vue.js projects. Whether you’re building a calculator or any other web application that requires user interaction, Vue.js is a versatile and powerful framework to consider